Flutter WEB
How to integrate and use the Apps Panel Web SDK in your app
Integration
To use the Flutter WEB SDK, add the git repository in the pubspec.yaml.
dependencies:
flutter_web_appspanel:
git:
url: ssh://[email protected]:2222/sdk-mobile/sdkmobilewebflutter.git
ref: 1.0.0-beta.9
The web SDK is not a class with static members or functions, you need to instantiate it, keep a reference to it, and use this instance (you can do this with get_it for instance)
final _apSdk = AppsPanelWeb();
_apSdk.init(/* params */);
await _apSdk.request(/* params */);
The following code snippets will assume an existing _apSdk
You need to init the sdk using environment variables. Add the env files at the root of the project with the needed variable. You'll need one for each environment.
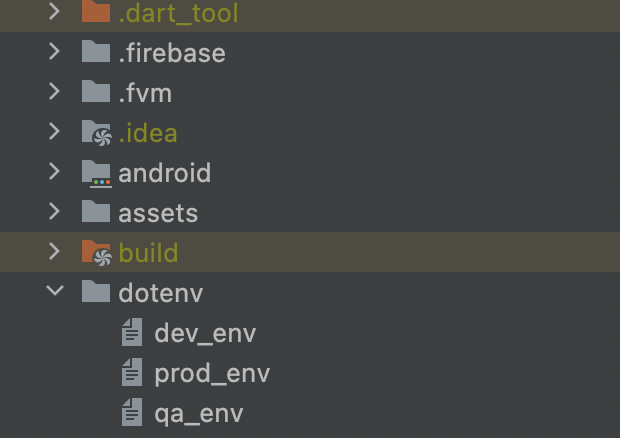
File namesDon't use file names starting with a dot, such as
.env
. The file will not be found by Flutter when deployed
URL_SDK="testsdkv5-dev.ap-sdk.com"
URL_BASE="testsdkv5-dev.ap-api.com"
APP_KEY="Pk18pGZHD8VbyhK7VaHFqA82"
APP_PRIVATE_KEY="YpehuTE0kVwreTyUVpjF9fNBaU6vOKua"
APP_NAME="testsdkv5-dev"
Then use a package like dotenv to read it and use variable in init
function.
await dotenv.load(fileName: "dotenv");
_apSdk.init(
envBaseUrl: dotenv.get(URL_BASE),
envSdkUrl: dotenv.get(URL_SDK),
envAppKey: dotenv.get(APP_KEY),
envAppName: dotenv.get(APP_NAME),
envPrivateKey: dotenv.get(APP_PRIVATE_KEY),
);
Text Manager
The downloaded content of the TextManager is saved in the LocalStorage of the browser.
Usage
You can use the widget or get the corresponding string.
AppsPanelTextManager(
tmKey: "apnl_key",
textStyle: const TextStyle(
fontSize: 35,
color: Colors.black,
),
align: TextAlign.center,
isSelectable: true, // will set text selectable and use specific cursor,
cursor: SystemMouseCursors.click // use selected cursor is isSelected is set to false
),
_apSdk.getTextManager(key);
Arguments
You can pass param arguments
to AppsPanelTextManager()
and getTextManager()
to replace pattern.
// today = "Today it's ##DAY##, it's ##HOUR##"
_apSdk.getTextManager("today", {"DAY": "Monday", "HOUR": "14h"});
// returns "Today it's Monday, it's 14h"
AppsPanelTextManager(
tmKey: "today",
arguments: {"DAY": "Monday", "HOUR": "14h"}
),
Web service requests
Like on mobile, you can use the SDK to make web service requests.
Future<List<Model>?> getListOfModels() async {
final response = await _apSdk.request(
route: "/path",
method: AppsPanelMethod.get,
sendToken: true,
);
if (response is RequestSuccessArray) {
final list = List.castFrom(req.data).map((e) => Model.fromJson(e)).toList();
return list;
} else if (response is RequestError) {
log("ERROR : ${response.message}, ${response.statusCode}, ${response.data}");
return null;
} else {
log("ERROR : ${response.runtimeType}");
}
}
KPI
You can send KPI for events KpiType.event
and views KpiType.view
.
_apSdk.sendKpi(KpiType.view, tag);
Updated about 2 months ago