Getting Started
How to integrate the Apps Panel SDK bridge in your app
The Apps Panel SDK is made to work with the Apps Panel Back Office. It provides a set of tools making your development easier and allowing access the features developed by Apps Panel.
The following information explain how to get started by implementing the SDK in your app.
The Flutter part of the Apps Panel SDK is not exactly an SDK on its own, it is mostly a bridge which communicates with the native iOS and Android SDKs.
Flutter dependency
In order to use the Apps Panel SDK features, add this to your pubspec.yaml
, you need to have access to https://gitlab.apnl.tech/sdk-mobile/sdkmobile-flutter.
dependencies
flutter_appspanel
git
url ssh //git@gitlab.apnl.tech 2222/sdk-mobile/sdkmobile-flutter.git
ref1.0.4
If you have not been given access to this repository, either ask for it if you can or if you've been given access to the source code without access to the repository, add a local dedendency
dependencies
flutter_appspanel
path ./path/to/local/sdk
Android installation
- Add all SDK environments configurations from product flavors settings :
android {
flavorDimensions "env"
productFlavors {
dev {
dimension "env"
buildConfigField "String", "AP_SDK_NAME", "\"myproject-dev\""
buildConfigField "String", "AP_SDK_KEY", "\"AbCdEfGhIjKlMnOpQrStUvWxYz\""
buildConfigField "String", "AP_SDK_PRIVATE", "\"AbCdEfGhIjKlMnOpQrStUvWxYz\""
resValue "string", "app_name", "My Project DEV"
applicationIdSuffix ".dev"
}
qa {
dimension "env"
buildConfigField "String", "AP_SDK_NAME", "\"myproject-qa\""
buildConfigField "String", "AP_SDK_KEY", "\"AbCdEfGhIjKlMnOpQrStUvWxYz\""
buildConfigField "String", "AP_SDK_PRIVATE", "\"AbCdEfGhIjKlMnOpQrStUvWxYz\""
resValue "string", "app_name", "My Project QA"
applicationIdSuffix ".qa"
}
prod {
dimension "env"
buildConfigField "String", "AP_SDK_NAME", "\"myproject-prod\""
buildConfigField "String", "AP_SDK_KEY", "\"AbCdEfGhIjKlMnOpQrStUvWxYz\""
buildConfigField "String", "AP_SDK_PRIVATE", "\"AbCdEfGhIjKlMnOpQrStUvWxYz\""
resValue "string", "app_name", "My Project"
}
}
}
- Create a MainApplication.kt file inside kotlin/com/myproject/app/ folder if not yet present and complete code as below :
package com.myproject.app
import android.content.Context
import android.util.Log
import io.flutter.app.FlutterApplication
import com.appspanel.flutter_appspanel.FlutterAPLocalConfiguration
import com.appspanel.flutter_appspanel.FluttersAppsPanelModuleBuilder
class MainApplication : FlutterApplication() {
override fun attachBaseContext(base: Context) {
super.attachBaseContext(base)
val appsPanelConfiguration = FlutterAPLocalConfiguration(
BuildConfig.AP_SDK_NAME,
BuildConfig.AP_SDK_KEY,
BuildConfig.AP_SDK_PRIVATE
)
Log.i("JME", "SDK install...")
FluttersAppsPanelModuleBuilder.init(this, appsPanelConfiguration);
}
}
- Next, you have to add
android:name=".MainApplication"
to your AndroidManifest.xml.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.myproject.app">
<application
android:name=".MainApplication"
android:label="My project"
android:icon="@mipmap/ic_launcher">
iOS configuration
Refer to iOS Getting started (v5) :
and Création app iOS
If you've followed #Création des configurations and #Variables de compilation then you should be able to configure the SDK on every environment by doing
#if DEV
try! AppsPanel.shared.configure(
withAppName: "myproject-dev",
appKey: "AbCdEfGhIjKlMnOpQrStUvWxYz",
privateKey: "AbCdEfGhIjKlMnOpQrStUvWxYz"
)
#elseif QA
try! AppsPanel.shared.configure(
withAppName: "myproject-qa",
appKey: "AbCdEfGhIjKlMnOpQrStUvWxYz",
privateKey: "AbCdEfGhIjKlMnOpQrStUvWxYz"
)
#elseif PROD
try! AppsPanel.shared.configure(
withAppName: "myproject-prod",
appKey: "AbCdEfGhIjKlMnOpQrStUvWxYz",
privateKey: "AbCdEfGhIjKlMnOpQrStUvWxYz"
)
#endif
Flutter
Add this at the launch of the app (in main.dart for example)
AppsPanel.init()
Build & run
Create a build configuration and specify your environment from the gradle build variant by adding arguments.
Corresponding commands :
flutter run --flavor=dev
flutter run --flavor=qa
flutter run --flavor=prod
For more information about Android specifications refer to the Android Getting Started (v5) documentation available from the link below :
https://appspanel.readme.io/docs/android-getting-started
Test
To be sure your SDK is correctly implemented check the back office web services logs to verify SDK requests at application startup.
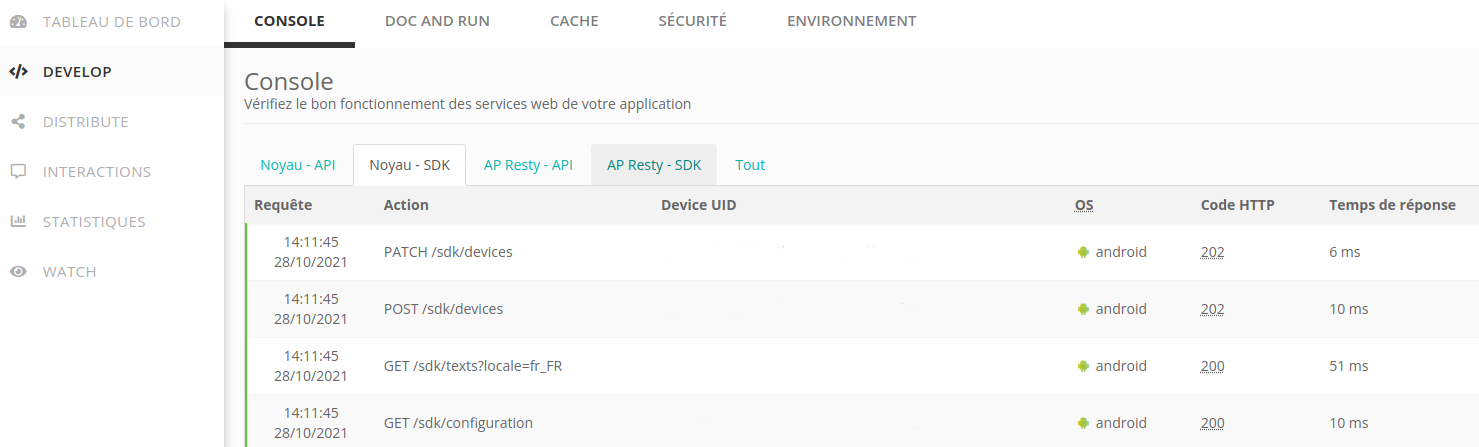
Moreover, you can check Logcat dev logs with "JME" tag :
2021-10-28 17:08:34.767 24966-24966/com.myproject.app.dev I/JME: SDK install
2021-10-28 17:08:34.767 24966-24966/com.myproject.app.dev D/JME: setConfiguration OK
2021-10-28 17:08:35.248 24966-24966/com.myproject.app.dev D/JME: initSDK
2021-10-28 17:08:35.248 24966-24966/com.myproject.app.dev D/JME: pluginBinding OK
2021-10-28 17:08:35.248 24966-24966/com.myproject.app.dev D/JME: APSDK.install()
2021-10-28 17:08:35.772 24966-25092/com.myproject.app.dev D/JME: APCrashManager init
2021-10-28 17:08:35.781 24966-25092/com.myproject.app.dev D/JME: postDevice
2021-10-28 17:08:35.787 24966-24966/com.myproject.app.dev D/JME: patchDevice
2021-10-28 17:08:37.258 24966-24966/com.myproject.app.dev D/JME: textManager
Updated about 2 months ago