Getting Started (v5) HMS
How to integrate the Huawei Mobile Services version of Apps Panel Android SDK in your apps ?
The Apps Panel Android SDK is made to work with the Apps Panel Back Office. It provides a set of tools making your development easier and permitting to access all the features developed by Apps Panel.
The following information explain how to get started by implementing the SDK in your app.
Add the SDK
First, to use the Apps Panel SDK, your application min SDK version must be set to API 19 minimum.
defaultConfig {
minSdkVersion 25
...
}
In order to find the Apps Panel SDK dependency, you need to add the maven repository in the build.gradle file of the project.
allprojects {
repositories {
//...
maven {
url 'https://repository.appspanel.com/repository/apnl-public-android-sdk/'
credentials {
username 'guest'
password 'CNONt0CkRzgqLpaEN92p'
}
}
}
}
The second step consist in adding the dependency in the build.gradle of your application.
dependencies {
//...
implementation 'com.appspanel.android:sdk:5.3.2-hms'
}
Configuration
In your Application
class ou can configure the SDK by two way :
First way you have to pass your configuration at the install
method. Call it by overriding protected void attachBaseContext(Context base)
in your Application class as following :
class MainApplication : Application() {
var appsPanelConfiguration = APLocalConfiguration(
"AppName",
"AppKey",
"PrivateKey",
"HmsAppId"
)
override fun attachBaseContext(base: Context) {
super.attachBaseContext(base)
APSDK.install(this@MainApplication, appsPanelConfiguration, this)
}
}
A second way consist in extending your Application class by APApplication as below :
class MyApplication : APApplication() {
override fun getAppsPanelConfiguration(): APLocalConfiguration {
return APLocalConfiguration(
"AppName",
"AppKey",
"PrivateKey",
"HmsAppId"
)
}
}
As parameter of APLocalConfiguration you must provide these elements:
- AppName : the name of your app on the Apps Panel Back Office
- AppKey : the key corresponding to your app on the Apps Panel Back Office
- PrivateKey : the private key to secure your app
- HmsAppId : the HMS application id from the Huawei App Gallery
HMS App IDIt correspond to the Project ID from the Huawei developer console.
Project settings / General information / Project information / Project ID
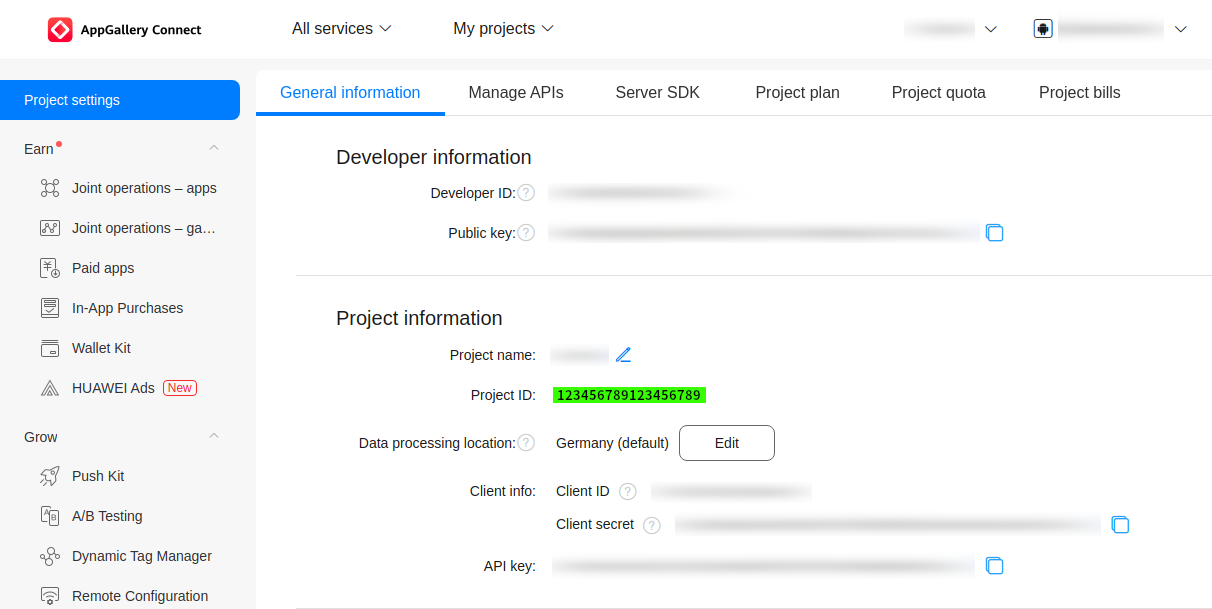
Best practice
A proper way to initialize the SDK is to use gradle parameters to manage several environments.
In your build.gradle file add the third attributes as below :
buildTypes {
debug {
//...
//SDK conf
buildConfigField "String", "AP_SDK_NAME", "\"my-project-dev\""
buildConfigField "String", "AP_SDK_KEY", "\"abcdefghijklmnopqr\""
buildConfigField "String", "AP_SDK_PRIVATE", "\"123456789ABCDE\""
buildConfigField "String", "HMS_APP_ID", "\"ABCDEFGHIJKLMNOPQRSTUVWXYZ\""
}
//...
}
And replace the Apps Panel SDK parameters :
var appsPanelConfiguration = APLocalConfiguration(
BuildConfig.AP_SDK_NAME,
BuildConfig.AP_SDK_KEY,
BuildConfig.AP_SDK_PRIVATE,
BuildConfig.HMS_APP_ID
)
HMS configuration file
Apply the HMS configuration to your project by adding the agconnect-services.json file downloadable from the HMS platform.
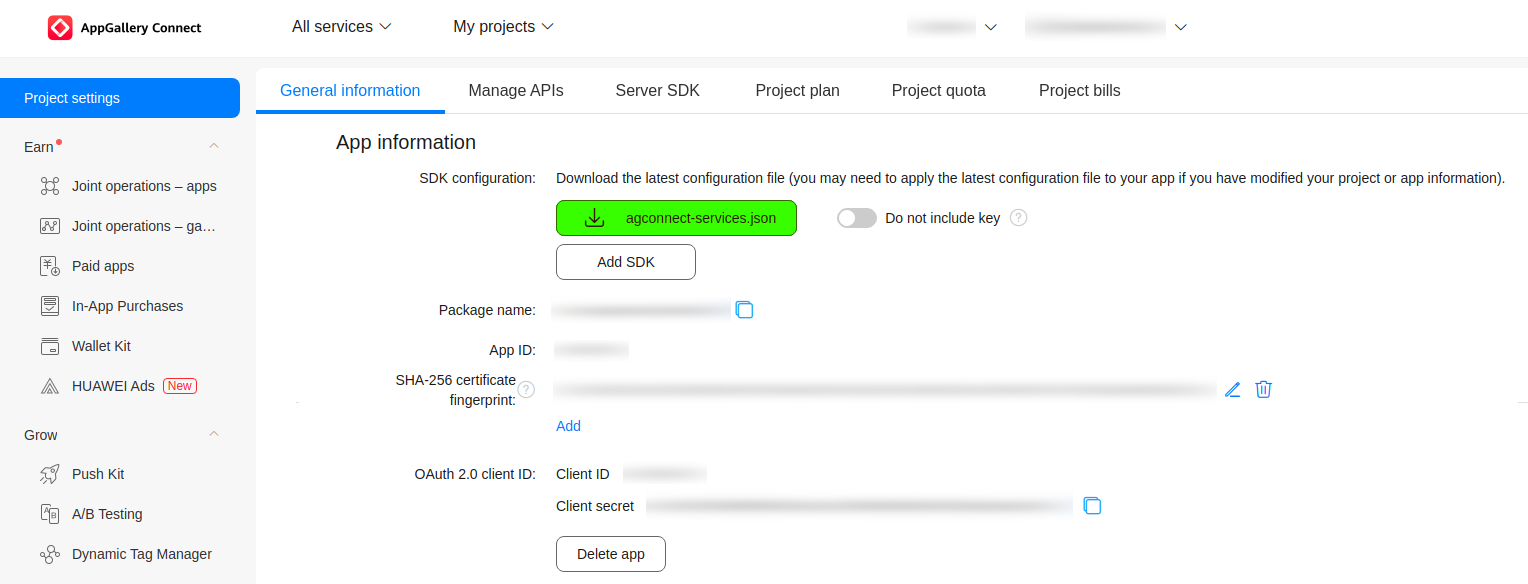
That's all folks
Congratulations, the SDK is now integrated, now you should be able to compile your application 🙌
Updated 30 days ago